How to use Bootstrap pagination in WordPress
Are you tired of the default WordPress pagination? Have you tried using Bootstrap pagination instead? This guide will show you how to implement Bootstrap pagination in your WordPress site and enjoy its sleek and customizable features. Follow these simple steps to get started.
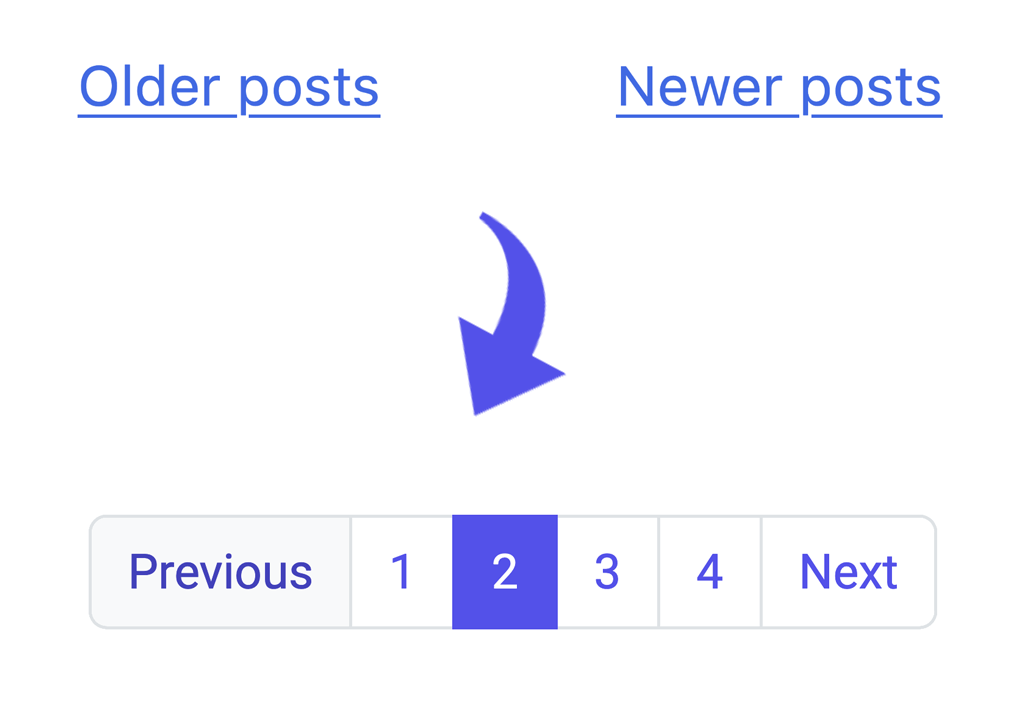
- Add Bootstrap to your theme
- Write the pagination function
- Replace the default pagination function with ours
Add Bootstrap to your theme
First, we need to add Bootstrap to the theme, so here we have some options.
Add from CDN
We can add Boostrap from CDN like this:
function custom_enqueue_styles() {
wp_enqueue_style(
'my_theme_bootstrap',
'https://cdn.jsdelivr.net/npm/bootstrap@4.5.3/dist/css/bootstrap.min.css'
);
}
add_action('wp_enqueue_scripts', 'custom_enqueue_styles');
Write the pagination function
The other way to add Bootstrap to our theme is to include it in our theme and then add it to the theme. Download Bootstrap
function custom_enqueue_styles() {
wp_enqueue_style(
'my_theme_bootstrap',
get_template_directory_uri() . 'path/to/bootstrap.css',
array(),
'1.0.0'
);
}
add_action('wp_enqueue_scripts', 'custom_enqueue_styles');
Write the pagination function
Now we can write a PHP function to replace it with the default WordPress pagination.
function bootstrap_pagination( $echo = true ) {
global $wp_query;
$big = 999999999; // need an unlikely integer
$pages = paginate_links( array(
'base' => str_replace( $big, '%#%', esc_url( get_pagenum_link( $big ) ) ),
'format' => '?paged=%#%',
'current' => max( 1, get_query_var('paged') ),
'total' => $wp_query->max_num_pages,
'type' => 'array',
'prev_next' => true,
'prev_text' => __('Previous'),
'next_text' => __('Next'),
)
);
if( is_array( $pages ) ) {
$paged = ( get_query_var('paged') == 0 ) ? 1 : get_query_var('paged');
$pagination = '<ul class="pagination">';
foreach ( $pages as $page ) {
$page = str_replace('<a ', '<a class="page-link"', $page);
$page = str_replace('<span ', '<span class="page-link active"', $page);
$pagination .= "<li class='page-item'>$page</li>";
}
$pagination .= '</ul>';
if ( $echo ) {
echo $pagination;
} else {
return $pagination;
}
}
}
Replace the default pagination function with ours
It might differ in any themes that the pagination has been included, but we’re sure it is used in our blog page. So we open the index.php file in our theme’s root folder and replace the function below:
the_posts_pagination();
to:
bootstrap_pagination();
Now It’s ready to have a look at the pagination in our posts page.
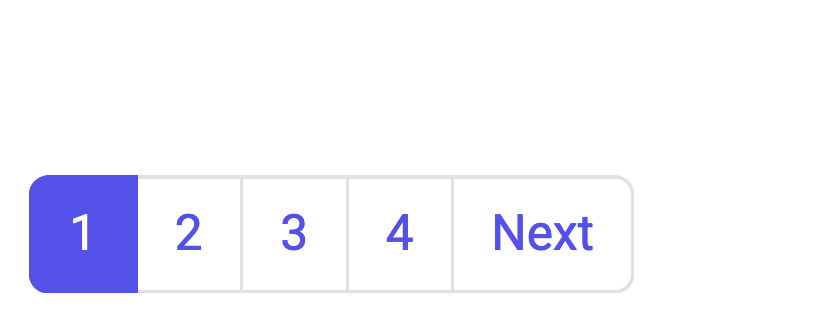
Now, you should have something similar to this. You can do many other customizations on the styles, but I preferred to stick to the simplest. So feel free to look at the bootstrap documentation and customize the pagination.